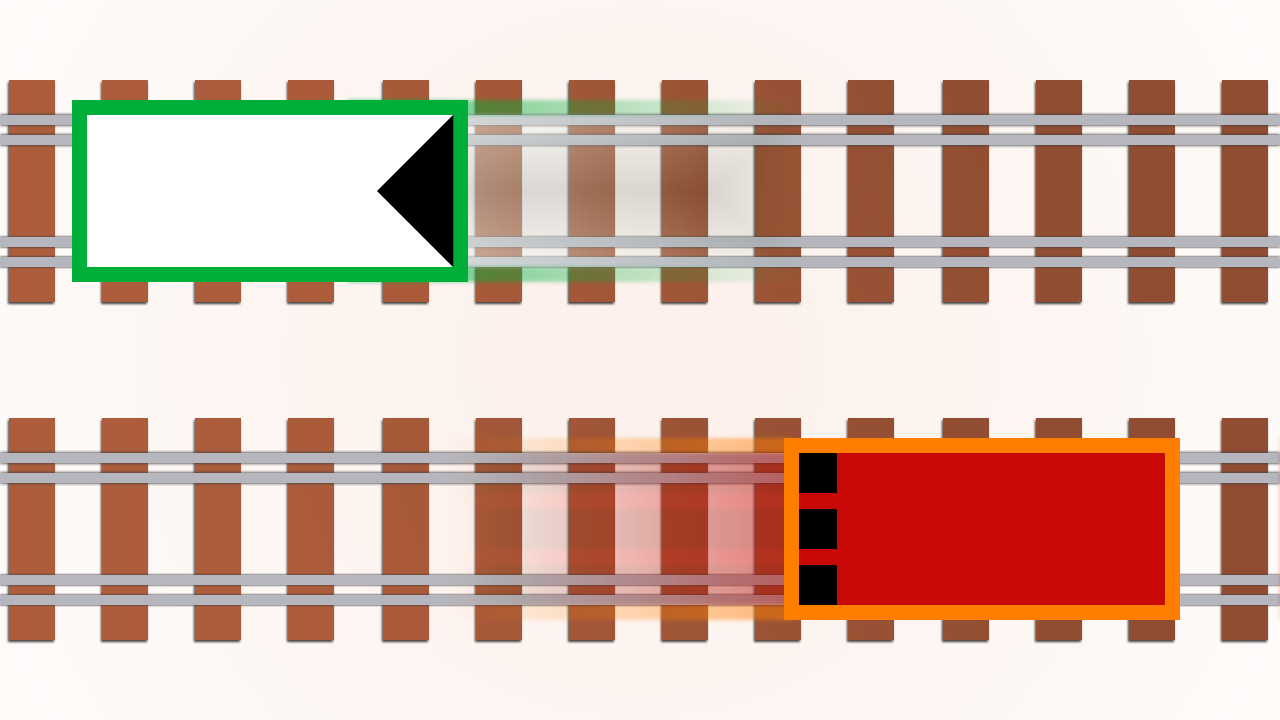
Finding The Fun In A Boring Task
As part of a recent contract job, I was tasked with creating around 150 distinct icons to represent trains in various statuses on an interactive map. This was a task that nobody was keen to pick up, as it involved long hours in an image editor.
I volunteered for the task to free up the full-time devs who have deeper domain knowledge of the product and are therefore better suited to some of the more nitty-gritty logic problems. I quite enjoy tinkering in image editors, but I knew I'd quickly get bored of this particular task regardless.
The boring task
As I settled in and started reading the requirements, I learned that the icons are mapped to a 4 character status code, and each of the digits (sometimes a combination) dictate various features of the icon. For instance, a certain character in the code being 'E' means a train is late, and a corresponding indicator is present in the icon.
Armed with this knowledge, I realised I could turn this menial task into a fun one by generating the icons on the fly based on a provided status code. At the same time, this approach would likely be faster to implement than manually creating the icons, and make icon changes much easier in the future.
Making the task fun
It proved to be quite simple to implement the icon generation. I couldn't avoid an image editor entirely; I had to create the unique components that can make up an icon (as transparent PNGs). Fortunately, these were mostly simple shapes.
Ultimately, the icon generation code looks something like this:
/**
* @param iconType the type of icon to generate, which includes width and height in px
* @param trainCode a 4 character code representing a train's status
* @return the generated icon
*/
public BufferedImage generateIcon(IconType iconType, String trainCode) {
List<BufferedImage> iconComponents = Arrays.asList(
getBodyBackgroundImage(iconType, trainCode),
getBodyImage(iconType, trainCode),
// more components...
);
BufferedImage result = new BufferedImage(iconType.getWidth(), iconType.getHeight(),
BufferedImage.TYPE_INT_ARGB);
// Stack the components together to create the final image
for (BufferedImage iconComponent : iconComponents) {
result.getGraphics().drawImage(image, 0, 0, null);
}
return result;
}
The takeaway
There's caching and some application-specific warts to contend with, but this blog post isn't really about the implementation of this feature.
At its essence, the icon generation was quick to implement and resulted in an elegant, fun solution to a boring problem. I put this post together to remind myself (and you, if you're reading!) that the "fun-ness" of a task can have very real implications for the quality and functionality of the end result. I've found that I'm vastly more productive when working on something I enjoy, and this solution saved time and delivered a better result in the end.
By transforming a boring task into a fun one, you can improve your productivity and have a good time along the way!